Creating a working login form with encrypted password authorization
In this documentation, we will create a login form through HTML and CSS to log in to a different page using Python. We will also encrypt a password, making the login process safe.
Creating a login form
Create an index.html file and create a login form. The following is the code for a simple login form created using Bootstrap HTML and CSS.
In this code, the name attribute in the input tag is the most important. We will use these names in our Python file to access the values of the input fields.
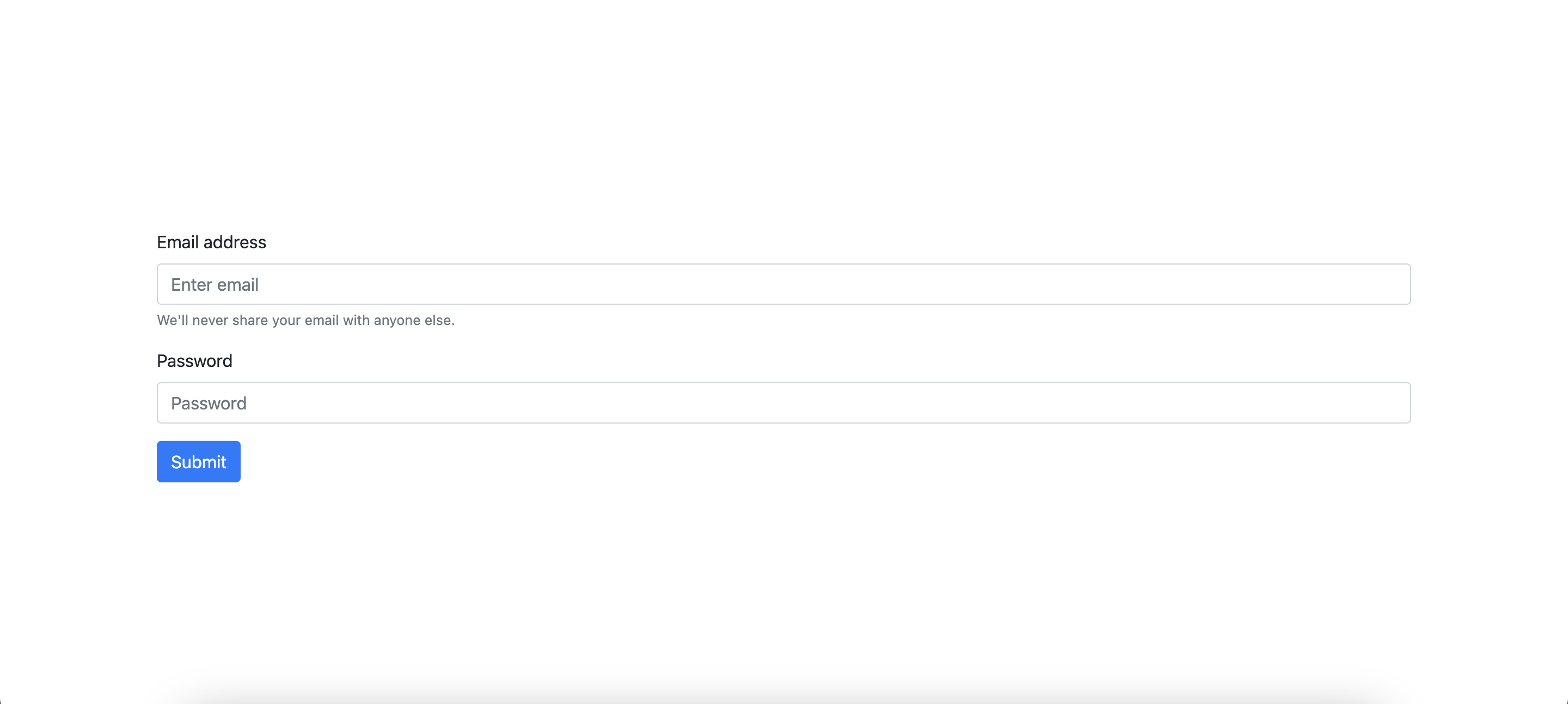
Arranging the files
Next, we will set up our file tree to interlink our code according to Python's Flask library's convention. Create two folders—templates and static—within the parent folder and an independent main.py Python file in the parent folder. Move the index.html file into the templates folder and any other non-HTML file (including CSS, JS, etc.) into the static folder. This is how your arrangement should look like.
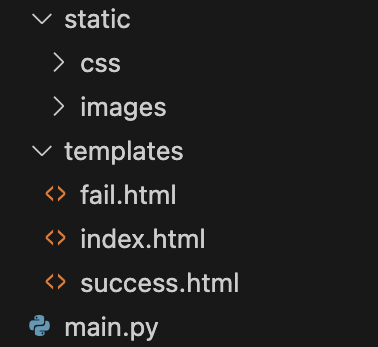
Create two HTML files within the templates folder with the titles success.html and fail.html. If the login fails, fail.html will be programmed to load. If login passes, success.html will load. Create another file called auth_crypt.txt, which will contain the encrypted password.
main.py
Now, we program the brain of the code. For that, we will use the boilerplate for Flask framework, a framework in Python that works at the intersection of Python and HTML and is used to program the back end of a website.
Here is the code:
import bcrypt
ENCRYPTED_PATH = 'auth_crypt.txt'
app = Flask(__name__)
def authenticate(entered_password):
saved_hash = fs.read()
fs.close()
enteredBytes = entered_password.encode('utf-8')
result = bcrypt.checkpw(enteredBytes, eval(saved_hash))
return result
def say_hello():
@app.route("/", methods=['GET', 'POST'])
def receive_data():
username = request.form.get("username")
password = request.form.get("password")
if authenticate(password) and username == 'Ved Panse':
The say_hello function returns the index.html page when the program is booted. Next, when the login form is filled, the main.py program will receive a post function. For that, the get_data function receives the data from the form and extracts the text content of the input fields using their name tag.
Here, the email id is stored in the variable name email_id and password, under the password variable. The authenticate function is used to authorize the password. Use it to encrypt the password that you want to set and store the encrypted password in the auth_crypt.txt file.
Lastly, within the get_data function, we call the authenticate function to validate the password entered. If the password is correct, we return success.html. If the password is incorrect, we return fail.html. This way, we have created a working login form that works by running main.py and visiting the link on which it starts running.